Functions Homework Help
Now we are moving to the higher level of C Language.
What is a Function?
A Function is a block of statements that performs a specific task. We can think of main() in the C Program as a Function which executes the entire program.
{`The syntax of writing a Function is as follow:
return_type function_name(argument_1, argument_2, ….)
{
//Block of statements;
return return_value;
}`}
When a Function doesn’t return anything, it is written as Void Type. For example:
#include <stdio.h>
#include <conio.h>
void display();
void main()
{
printf(“The display function will be executed below this.”);
display();
getch();
return;
}
void display()
{
printf(“\nWelcome to the display function.”);
}
In the above example you have seen that we have written the first line of Function just before the main Function. That is called as the “Function Prototype Declaration”. A Prototype Declaration of Function is a must.
There can any number of Functions in a C Program. The main Function calls the other Functions in the program. One Function can call other Functions too.
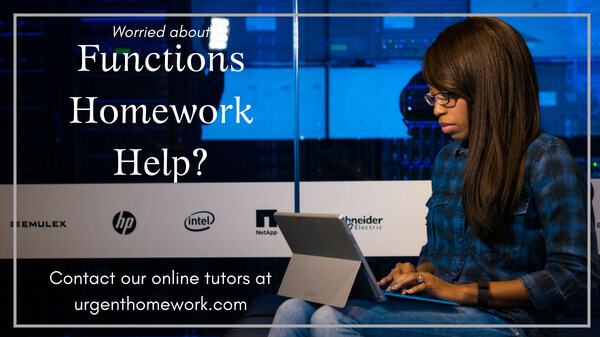
Function in C Language Example
#include <stdio.h>
#include <conio.h>
void display1();
void display2();
void main()
{
printf(“This example shows the calling of one function by another.”);
display1();
return;
}
void display1()
{
printf(“\nWe are in display1 function. We are going to call display2.”);
display2();
return;
}
void display2()
{
printf(“\nThis is display2 function.”);
return;
}