Inheritance
- Inheritance is the process by which new classes called derived classes are created from existing classes called base classes.
- The derived classes have all the features of the base class and the programmer can choose to add new features specific to the newly created derived class.
Advantages of Inheritance
- Reusability of Code
- Saves Time and Effort
- Faster development, easier maintenance and easy to extend
- Capable of expressing the inheritance relationship and its transitive nature which ensures closeness with real world problems.
Base & Derived Classes:
A class can be derived from more than one classes, which means it can inherit data and functions from multiple base classes. A class derivation list names one or more base classes and has the form:
class derived-class: access-specifier base-class
Where access is one of public, protected, or private.
For example, if the base class is MyClass and the derived class is sample it is specified as:
class sample: public MyClass
The above makes sample have access to both public and protected variables of base class MyClass.
Example of Inheritance
Consider a base class Shape and its derived class Rectangle as follows: class Shape { public: void setWidth(int w) { width = w; } void setHeight(int h) { height = h; } protected: int width; int height; }; Derived class class Rectangle: public Shape { public: int getArea() { return (width * height); } }; int main(void) { Rectangle Rect; Rect.setWidth(5); Rect.setHeight(7); Print the area of the object. cout << "Total area: " << Rect.getArea() << endl; return 0; }
When the above code is compiled and executed, it produces following result:
Total area: 35
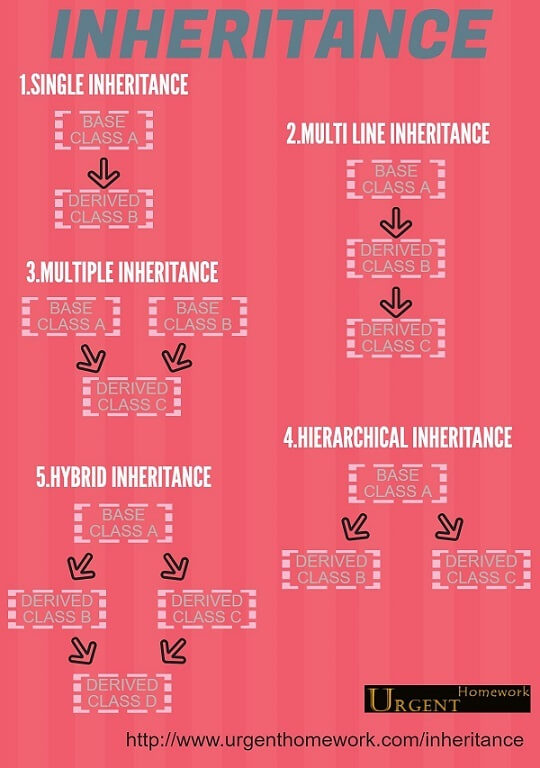
Access Control and Inheritance:
A derived class can access all the non-private members of its base class. Thus base-class members that should not be accessible to the member functions of derived classes should be declared private in the base class. We can summarize the different access types according to who can access them in the following way:
MODE OF VISIBILITY | PUBLIC | PROTECTED | PRIVATE |
---|---|---|---|
Same class | yes | yes | yes |
Derived classes | yes | yes | no |
Outside classes | yes | no | no |
1. Public Inheritance: When deriving a class from a public base class, the base class become public members of the derived class and protected members of the base class become protected members of the derived class. A base class's private members are never accessible directly from a derived class, but can be accessed through calls to the public and protected members of the base class.
2. Protected Inheritance: When deriving from a protected base class, public and protected members of the base class become protected members of the derived class.
3. Private Inheritance: When deriving from a private base class, public and protected members of the base class become private members of the derived Class
Types of Inheritance
1. Single class Inheritance: Single inheritance is the one where you have a single base class and a single derived class.
2. Multilevel Inheritance: In Multi level inheritance, a subclass inherits from a class that itself inherits from another class.
3. Multiple Inheritance: In Multiple inheritances, a derived class inherits from multiple base classes. It has properties of both the base classes.
4. Hierarchical Inheritance: In hierarchial Inheritance, it's like an inverted tree. So multiple classes inherit from a single base class.
5. Hybrid Inheritance: It combines two or more forms of inheritance .In this type of inheritance, we can have mixture of number of inheritances but this can generate an error of using same name function from no of classes, which will bother the compiler to how to use the functions. Therefore, it will generate errors in the program. This has known as ambiguity or duplicity.