CMIS 102 Hands-On Lab Week 6
Week 6 Overview
This hands-on lab allows you to follow and experiment with the critical steps of developing a program including the program description, analysis, test plan, design and implementation with C code. The example provided uses sequential, repetition statements and nested repetition statements.
Program Description
This program will calculate the average of 3 exams for 5 students. The program will ask the user to enter 5 student names. For each of the students, the program will ask for 3 exam scores. The average exam score for each student will be calculated and printed.
Interaction
Starting with this project, the interactions between the program and the user are getting complicated enough to justify a new section before the Analysis section – a section which will eventually become the User’s Guide. This section is a rather detailed presentation of how the program and the user will interact. A little reflection should make it apparent that neither the Analysis nor the Test Plan can really proceed without an understanding of how the program will work from the user’s perspective.
At the moment, the exact interaction is not defined by the Program Description, so this is our chance to make that interaction clear and convenient, and we will make choices. In this case, we choose to enter the 3 numbers, followed by a name. There should be spaces between the numbers and the between the third number and the student’s name. For this program, we will allow all the information to be on a single line. Here is an example, the prompt in black, the user input in red:
Enter 3 grades and the student’s name: 88.76 93 98.22 Mary
Analysis
New concept: reading, storing and printing a string input from the user. This is going to require research or an example.
Required storage:
- float data: new grade, sum of grades, average of those grades
- string data: student’s name Math:
- sum = sum + new grade
- average = sum / 3 Example of one student:
- input: 70 80 90 Jane
- sum = 0
- sum = sum + 70
- sum = sum + 80
- sum = sum + 90
- sum is 240 at this point
- average = 240 / 3 = 80
Output for one student
- Average for Jane is 80
Looping:
- for 5 students o reset sum o for 3 grades
- get grades o get name o compute average o print result for one student
- end for 5 students
Test Plan
To verify this program is working properly the input values shown in the table below could be used for testing.
Comments:
- Can we make sure that the output is exactly two digits right of the decimal point? Yes!
- Can we average ANY 3 numbers? Yes!
- How should this code behave if there are any deviations from the specified input format? Any good answer is going to involve rather complicated and advanced code – beyond anything we want to explore in this class.
- Can the student’s name have any spaces or special characters? Not really.
- Can the fields be separated by more than one space? You should check this through experiments.
Test Case |
Input |
Expected Output |
1 |
1 1 1 a 2 2 2 b 100 200 300 c -1 -2 -3 d 10 20 30 e |
Average for a is 1.00 Average for b is 2.00 Average for c is 200.00 Average for d is -2.00 Average for e is 20.00 |
2 |
90.0 80.0 100.0 John 80.0 70.0 90.0 Jim 70.0 100.0 100.0 Joe 100.0 95.0 91.0 Sally 30.0 54.0 68.0 Sam |
Average for John is 90.00 Average for Jim is 80.00 Average for Joe is 90.00 Average for Sally is 95.33 Average for Sam is 50.67 |
Pseudocode
// This program will calculate the average // of 3 exams for 5 students // Declare variables Declare StudentName as String Declare ExamValue, Sum, Avg as Float // Loop through 5 Students For (students=0; students <5 ; students++) // reset Sum to 0 Set Sum =0.0 Print “Enter 3 grades and student Name” // Nested Loop for Exams For (exams=0; exams < 3; exams++) Print “Enter exam grade: \n” Input ExamValue Set Sum = Sum + ExamValue End For Set Avg = Sum/3.0 Input StudentName Print “Average for “ + StudentName + “ is “ + Avg End For
C Code
The following is the C Code that will compile in execute in the online compilers.
// C code // This program will calculate the average // of 3 exams for 5 students. // Developer: Faculty CMIS102 // Date: Jan 31, XXXX #include < stdio.h > int main(void) { // variable declarations: char name [100]; float sum, grade, average; int students, exams; // loop through 5 students for (students = 0; students < 5; students++) { printf ("Enter 3 grades and student name: "); sum = 0; for (exams = 0; exams < 3; exams++) { // float uses %f, double uses %lf scanf ("%f", &grade); sum = sum + grade; } // end for each exam average = sum / 3; scanf ("%s", name); printf ("Average for %s is %.2f\n", name, average); } // end for each student printf("... Bye ...\n"); return 0; } // end main
Screen Shots:
Setting up the code panel in repl.it:
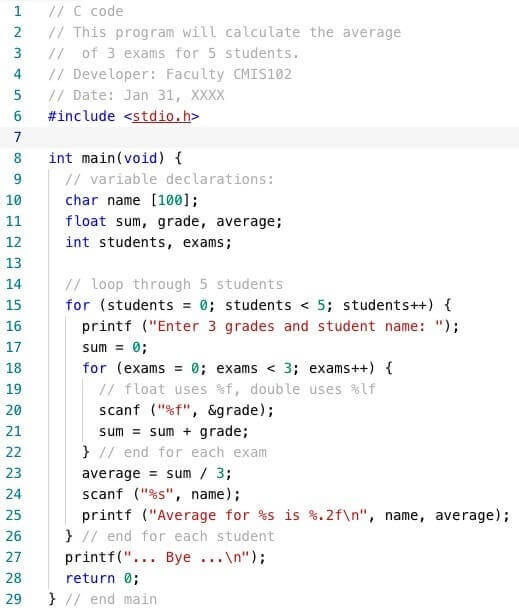
Note the Student and ExamValues for this run were:
- 0 80.0 100.0 John
- 0 70.0 90.0 Jim
- 0 100.0 100.0 Joe
- 100.0 95.0 91.0 Sally
- 0 54.0 68.0 Sam
You can change these values to any valid integer values to match your test cases.
Results from running the programming at repl.it
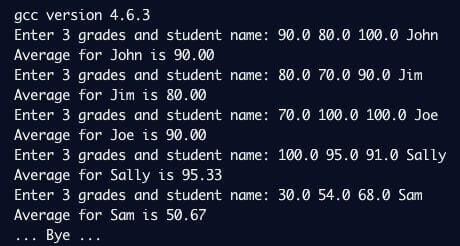
Learning Exercises for you to complete
- Demonstrate you successfully followed the steps in this lab by preparing screen captures of you running the lab as specified in the Instructions above.
- Modify the code to be able to input any number of students (i.e. the loop is controlled by the user input). You will still only have 3 exams for each student. Support your experimentation with screen captures of executing the new code.
- Prepare a new test table with at least 3 distinct test cases listing input and expected output for the new code you created allowing the input of any number of students.
- What is the line of code doing? char StudentName[100]; (Hint: We haven’t covered arrays, but a String can be thought of as an array of characters) ?
- What would happen if you moved the Set Sum = 0.0 from inside the for loop to right after the declaration. For example:
// Declare variables
Declare StudentName as String
Declare ExamValue, Sum, Avg as Float
// Initialize Sum Set Sum = 0.0;
Support your experimentation with screen captures of executing the new code.
- (Optional and challenging) How could you modify the input protocol and the code to allow the user to enter more than 3 grades per student?
- (Optional and even more challenging) How could you modify this code to allow the user to enter a different number of grades for each student?
- (Optional and even more challenging) How could you modify this code to allow the user to enter a weight for each grade, as in Lab 5?
Grading guidelines
Submission |
Points |
Demonstrates the successful execution of this Lab within an online compiler. Provides supporting screen captures. |
30 |
Modifies the code to be able to input any number of students (i.e. the loop is controlled by the user input). You will still only have 3 exams for each student. Supports your experimentation with screen captures of executing the new code. |
20 |
Prepares a new test table with at least 3 distinct test cases listing input and expected output for the new code you created allowing the input of any number of students. |
10 |
Describes what the char array line is doing. |
10 |
Describes what would happen if you moved the Set Sum = 0.0 from inside the for loop to right after the declaration. Be sure to provide screen captures to document your analysis and results. |
20 |
Document is well-organized, and contains minimal spelling and grammatical errors. |
10 |
Total |
100 |