C Programming Help
Function With Arguments/Parameters/Variables
There are two ways of calling a Function in C.
- Call by value
- Call by reference
Call by value means, we give some values as arguments to a Function and it returns some value. Suppose we have made a program to add two numbers:
{`int add(int a, int b)
{ ;
//return a+b;
}`}
Now we call this Function in our main program,
add(m,n);
where m and n are the two integers defined in our main Function.
What will happen here ?
The value of m and n will be copied in a and b and the Function will be executed. Hence after the execution of statement if you want to access the value m or n, it wont change, as the value has been copied.
This is the example of call by value. We can make this more clear by using swap Function:
Let us suppose that we have defined a Function swap which takes two integers as argument and swaps there values:
void swap(int a, int b)
{
printf(“\n The value of a is: %d”,a);
printf(“\n The value of b is: %d”,b);
int temp;
temp = a;
a = b;
b = temp;
printf(“\n The value of a is now: %d”,a);
printf(“\n The value of b is now: %d”,b);
return;
}
Now we call this Function in our main program using:
int x,y;
x = 10;
y = 20;
swap(x,y);
printf(“\n The value of x is now: %d”,x);
printf(“\n The value of y is now: %d”,y);
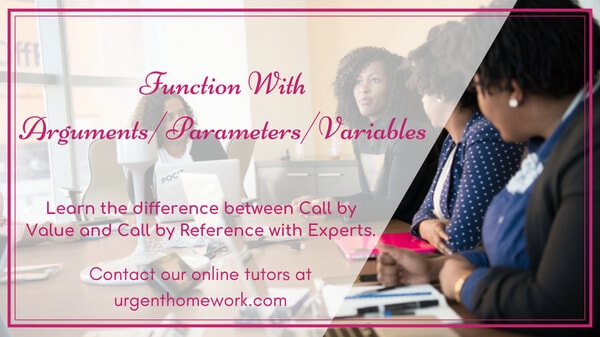
Tell me what do you expect the answer should be:
I think most of you must be thinking as we have swapped the values of x and y hence x should become 20 and y should be 10.
But CAREFUL !! You are making a mistake. As I told you when you call a function by value, the arguments are copied only. Hence the values of x and y wont change.
This is where comes the another part of game. “CALL OF FUNCTION BY REFERENCE”. We’ll discuss this in great detail when we’ll learn the chapter pointers.
In Call by reference, we give the address of the variables and the Function performs tasks directly on the variable and not on the copied values.