Structures Homework Help
Structures are among the most important items in C Programming Language. It is difficult to program using only Int, Float and Char. In real life Programming, instead of these data types we use a data type which includes these data types. For example, to describe a car we need to tell its Manufacturer, Model no, Color, and Number. We can think of car as a Structure with following definition:
car
{
char manufacturer[50];
int model_no;
char color[10];
int number;
}
A Structure is a collection of Variables under a single name. These Variables can be of different types and each has a name which is used to select it from the Structure. A Structure is a convenient way of grouping several pieces of related information together.
A Structure can contain any valid data types like Int, Char, Float even Arrays and other Structures. Each Variable in Structure is called a Structure member.
Defining Structure
To define a Structure, you can use Struct Keyword. Here is the common syntax of Structure Definition:
struct struct_name{ structure_member };
The name of Structure is followed the rule of Variable Name.
struct book
{
char name;
float price;
int pages;
};
To define instances of book type Structure, we use following:
struct book b1, b2, b3;
A Structure could be complex if it contains other Structures inside itself.
Accessing Members
We use .(dot) to access the element/member of a Structure.
For example, b1.name will return the name of b1 book.
Where we have a Pointer to a Structure we could dereference the Pointer and then use dot as a member selector. This method is a little clumsy to type. Since selecting a member from a Structure Pointer happens frequently, it has its own operator -> which acts as follows. Assume that book_ptr is a Pointer to a Structure of type book. We would refer to the name member as:
Book_ptr -> nameType Def Keyword
To make your source code more concise, you can use typedef keyword to create a synonym for a Structure. For example:
typedef struct
{
char name[50];
int roll_no;
} student;
student s1, s2;
After using typedef keyword we don't need to use struct word all the time.
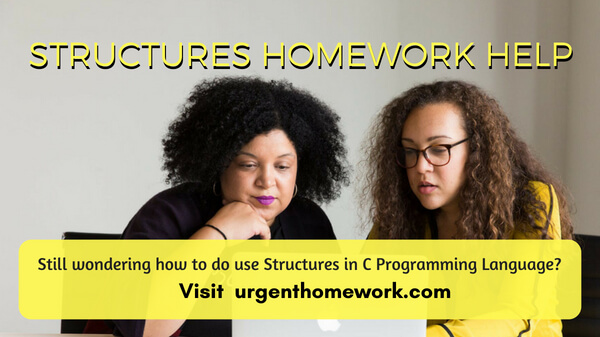
Size Of Function
sizeof Function is used to determine the size of any Data-Type. As a Structure is also a Data-Type it works with Structure as well. For example:sizeof(student) = 50 + 4 = 54 Bytes
where student is the Structure defined above.