Pointers Homework Help
If you really want to be good in C Programming, Pointer is the must. In this chapter we’ll learn in detail about the Pointers and its usage in C Programming.
When we declare a Variable we inform the compiler of two things, the name of the Variable and the type of the Variable. For example, we declare a Variable of type integer with the name i by writing:
Int i = 5;
What happens when the compiler sees this?
The Compiler sets aside 4 bytes of memory to hold the value of the integer. It sets up a symbol table. In that table it adds the symbol i and the relative address in memory where those 4 bytes were set aside. The value 5 will be placed in that memory location reserved for the storage of the value of i. In a sense there are two values associated with the object i. One is the value of the integer stored there and the other the value of the memory location, i.e., the location of i.
A Pointer is a Variable that contains the memory location of another Variable. You start by specifying the type of data stored in the location identified by the Pointer. The asterisk tells the compiler that you are creating a Pointer Variable. Finally you give the name of the Variable.
Type * Variable Name
Example:
int *intptr;
float *fltptr;
The address that locates a Variable within memory is what we call a reference to that Variable. This reference to a Variable can be obtained by preceding the identifier of a variable with an ampersand sign (&), known as reference operator and which can be literally translated as "address of".
The & And * Signs
Consider the following statements:
int x,y;
int *ptr;
x = 2;
ptr = &x;
y = *ptr;
Now, I shall tell you what the meanings of these statements are. Firstly, we declared two integer Variables x and y and a Pointer to integer ptr. The * sign means we are declaring a Pointer that points to an integer. In other words, we can say that the Pointer ptr will always point to a memory location which will contain integer. After this, we assign x a value (2 in this case). Now in the next statement, ptr is assigned a memory address of x. This means now, the Pointer Variable ptr will point to the memory location which contains the Variable x. In the next statement, y=*ptr, we assign y a value which is equal to the value stored in the memory location pointed by ptr. i.e. value of x.
If this is confusing for you, I’ll make it simple. When we declare a Pointer, we always assign what type of values it will point to. This means, whether it will point to an integer, or a float or a char or a structure. Then we initialize our Pointer by giving this address of a memory location, this is done by & operator. &x means, address in memory where x is stored. Putting * before a Pointer will return you the value stored in that memory location.
Hence,
int x = 9;
*(&x) will give the value of x itself i.e. 9.
As &x will return the address and *(address) will return content at that address.
I hope this has cleared your doubts about & and * signs. If not, read this again.
#include <stdio.h>
#include <conio.h>
void main()
{
{//}int i, *intptr;
printf(“Enter some value of i: ”);
scanf(“%d”,&i);
intptr = &i;
printf(“\nThe value of i is %d, which is stored in %u memory address.”,i,&i);
printf(“\nThe value of i is %d, which is stored in %u memory address.”,*intptr, intptr);
getch();
return;
}
When you will execute this program, you’ll see that the outputs generated by second and third printf statements are the same. Now you know the reason.
Pointer Arithmetic
Pointers also follow the Arithmetic Operations as the normal Int or Float Variables follow but there is a slight difference.
Consider the following example:
int i, *ptr;
ptr = &i;
ptr = ptr + 1;
what do you think, what will happen ?
Int occupies 4 Bytes of space on memory. So, when we declare Int i, 4 Bytes of space will be allocated to i. Now, we assign ptr the address of that location.
Byte 0 | Byte 1 | Byte 2 | Byte 3 |
The values 50122, 50123 etc are the arbitrary assigned values to the memory location.
ptr contains the value 50122.
The operation ptr + 1 will move the Pointer to next memory location.
ATTENTION !!
Now, pointer will not point to 50123 rather it will point to 50122 + 4. As this is Integer Pointer and size of an Integer is 4 Bytes.
We will use this a lot when we will go to the chapter Arrays.
Call By Reference:
When we pass address to a Function the parameters receiving the address should be Pointers. The process of calling a Function by using Pointers to pass the address of the Variable is known as call by reference. The Function which is called by reference can change the values of the Variable used in the call.
{/* example of call by reference */}
{`#include <stdio.h> #include <conio.h> void myfunction (int* p, int* q) { int temp; temp = *p; *p = *q; *q = temp; return; } int main() { int x,y; x=20; y=30; printf("\n Value of x and y before function call = %d %d", x, y); myfunction(&x,&y); printf("\n Value of x and y after function call =%d %d", x, y); getch(); return; } `}
We will come back to Pointers again after we finish Array and Structure.
Topics in C Programming
- Data Type
- Input Output
- Formatting Of Output
- Arithmetic Operations
- Logical Operators
- Order Of Precedence
- Decision Control
- Loops
- Functions
- Passing Values
- Function Calls
- Recursion
- Pointers
- Structures
- Structures and Pointers
- Array
- File Handling In C
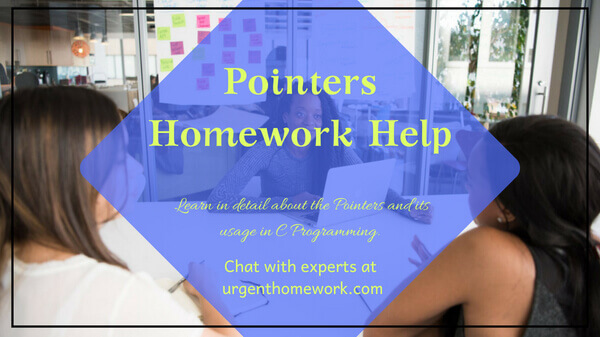