Help with OOPS in java
JAVA:
Java is the one of the high level, robust, object-oriented and secure programming language. It works on different platform like Windows, Mac, Linux, Raspberry Pi, etc. It is open source and free with highly secure. OOPs concept in java is very important to design systems in the object-oriented programming model.
OOP:
Object oriented programming aims to implement real-world entities like inheritance, hiding, and polymorphism in programming. The main aim of OOP is to bind together the data and the functions that operate on them.
OOPs Concept:
- Class
- Object
- Polymorphism
- Inheritance
- Encapsulation
- Abstraction
- Method
- Message Passing
Class:
A class is a user defined blueprint which contains the entire set of similar data and functions that the object possess. The class declarations can include following components
- Modifiers : A class can be public or has default access.
- Class name: The name should begin with a initial letter (capitalized by convention).
- Super class: The name of the class’s parent if any, preceded by the keyword extends. A class can only extend (subclass) one parent.
- Interfaces: The list of interfaces implemented by separated commas of the class. A class can implement more than one interface.
- Body: The class body surrounded by braces, { }.
{`class Home { String adds; String colour; double state; void openhouse() { //Write code here } void closehouse() { // body of the code } ... } `}
Objects:
An object consists of state and behaviour. In the real world we may see many objects like house, car, dog etc. the object of class is known as instance.
Objects have two characteristics: They have states and behaviours.
Examples of states and behaviours
Example:
Object : House
State : Address, Colour, Area
Behaviour : Open door, close door
The house has the state of address, colour, area and have the behaviour of open door, close door.
Initial object using new operator:
House h = new house(); // new operator
Polymorphism
Polymorphism means taking many forms. It is the capability of a variable, function or object to take on multiple forms. Single Function name perform different task is called function overloading.
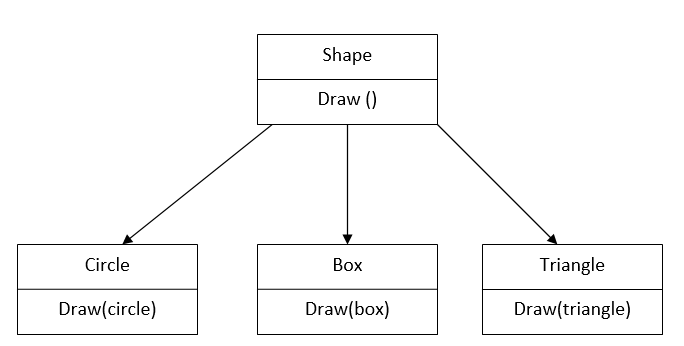
In this draw is same function name for different purpose. First draw function used for drawing a circle, the same used for box, the same used for the triangle.
Example:
{`public class shape { public void drawing() { System.out.println("Drawing circle using default diameter 1 cm."); } public void drawing(int diameter) { System.out.println("Drawing box using default diameter" + diameter +" cm."); } public void draw(int diameter, String color) { System.out.println("Drawing triangle using colour " +color+" and diameter" +diameter+" cm."); } } `}
Inheritance:
It is the process of where one object acquires the properties of another object. In oop, the concept of inheritance provides the idea of reusability; this means that we can additional features to an existing class without modifying it. This is possible by deriving a new class from the existing one. The new class will have the combined features of those classes.
Extend keyword:
The keyword extend used for inherits the properties of classes.
Example:
{`Class one { Body of class } Class two extends one { Body of class } `}
Encapsulation:
The wrapping up of data and methods into a single unit it is called encapsulation. These methods provide the interface between the object’s data and program. The insulation of the data from direct access by the program is called data hiding. It allows us to protect data store in class from system wide access.
The encapsulation of data in java done by Restricts direct access to data members (fields) of a class, Fields are set to private, Each field has a getter and setter method, Getter methods return the field, Setter methods let us change the value of the field
Example:
{`class Encapsulation { private int essn; private String eName; public int getEmpSSN(){ return essn; } public String getEmpName() { return eName; } public void setEmpName(String newValue) { eName = newValue; } public void setEmpSSN(int newValue){ essn = newValue; } } public class EncapsTest { public static void main(String args[]) { Encapsulation obj = new Encapsulation(); obj.setEmpName("Malar"); obj.setEmpSSN(13456); System.out.println("Employee Name: " + obj.getEmpName()); System.out.println("Employee SSN: " + obj.getEmpSSN()); } } `}
Output:
Employee Name: Malar
Employee SSN: 13456
The above example data members are private it cannot accessed directly.
Java Tutorials
- Environment setup
- Basic concept
- Object Classes
- Constructors
- OOPS in java
- data abstraction
- Variable Types
- Modifiers
- Operators
- Loop Controls
- Decision Making
- Strings
- Arrays
- Date and Time
- Methods in java
Java sample assignments
Programming Topics
- Ada
- Assembly Language
- AutoCAD
- BASIC
- Computer virus
- C Programming
- Euphoria
- Fortran Homework Help
- Game programming language
- Java Assignment Help
- JavaScript
- Java Servlets Help
- Machine Language
- Matlab
- Pascal
- Perl
- PHP
- Python
- Ruby
- Servlet Life Cycle
- Smalltalk
- SOAP
- Visual Basic
- COBOL
- Lisp
- Logo Help
- Plankalkul Help
- Prolog
- REBOL
- Rexx
- Scheme Help
- TCL
- ToonTalk Help